Abstract—The objective of the project is to create a simple Real Time Operating System (RTOS) for the
Cortex-M4 microprocessor by implementing a task scheduler and an Operating System API to make
ISR-OS interface. The system should be capable of handling multiple tasks with varying priorities and
should switch the tasks as that of in RTOS by round-robin algorithm, accommodating other system
Interrupt Service Routines (ISRs). The goal is to minimize the overhead on processor with efficient
system performance.
I. I NTRODUCTION
A mini RTOS is designed to handle multiple tasks with different priorities and system Interrupt
Service Routines (ISRs) by algorithms such as round-robin algorithm. One important aspect of
an RTOS is its context switching, which allows the system to save the current running task state
in stack and then switch to a higher-priority task when it is available. Tasks running in RTOS system
will be appear as shown in fig 1. Task priorities are used to determine the order in which tasks
are executed. The OS calls are implemented using SVC call, PendSV and SysTick interrupts. The
SVC and PendSV interrupt is used to perform context switching while the SysTick interrupt is
used to run the scheduler, ensuring that the highest priority task is always executed first. The goal of
this mini RTOS is to minimize overhead on the processor by efficient task scheduling.
A. Architecture
This section describes the architecture of this project. The preemptive task scheduler discussed
here needs to be implemented in ARM Cortex-M
microcontroller, specifically the TM4C123GH6PM. It uses the SVC, PendSV exception handler to
perform context switching between tasks. When a context switch is required, the current task’s context
is to be saved, and the context of the next task to be executed is restored. The pendsv function should
trigger a PendSV exception and request a context switch.
In addition to PendSv, It should consist of SysTick exception handler to periodically select the next task
to be executed based on its priority. we need to have a function for Systick Handler to update the
delay values of all waiting tasks and moves tasks that have completed their delay to the ready state.
If necessary, it need to set up a context switch by calling the pendsv function.
We need to set up a function to launch the Scheduler to first initialize the scheduler by dummy task and
then once it is set up we need to get the next task to be executed. The scheduler uses a simple round-
robin approach to break ties between tasks with the same priority. If no task is ready to run, the
scheduler is set to run the current task only.
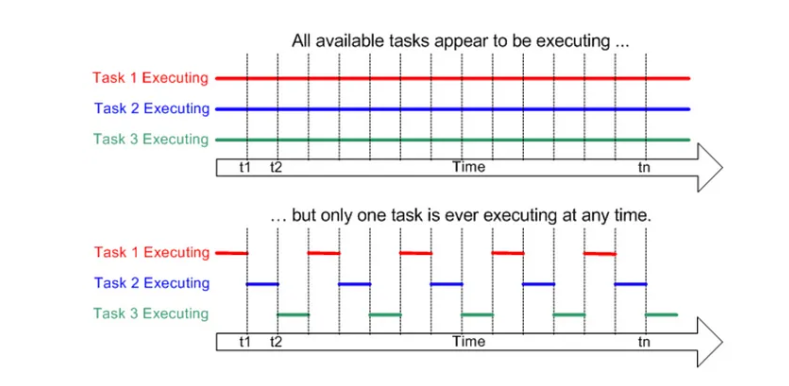
Fig. 1. Tasks scheduled by preemptive scheduler
B. Structure
The header file contains structures and functions related to an operating system (OS) for managing
tasks. The OS manages tasks using a priority-based scheduling algorithm. Here, we will discuss the
various structures that were created upto now and used in this file.
• Task State – This is used to define the state of a task. It has five values – running, wait, ready,
block, and suspended. These values are used to represent the current state of a task.
• TCB – This is a structure which represents a task control block (TCB). The TCB contains
information about a task such as its stack pointer, priority, delay, task IDs and the address of the task
function. This information is used by the OS to manage the task.
• PendSv setup – This is used to set up the PendSV exception. This exception is used by the
OS to perform a context switch.
• PendSv Handler – This function is the exception handler for the PendSV exception. It performs a
context switch by saving the current task’s contextand restoring the next task’s context that needs to
be executed.
• PendSv call – This function triggers the PendSv exception, which is used for context switching.
• Systick Handler – This function is the exception handler for the Systick timer interrupt. It is used
by the OS to keep track of time and perform time-based operations.
• LaunchScheduler – This function launches the scheduler, which is responsible for scheduling
tasks and performing context switches.
• MAX NUM OF TASKS and STACKSIZE are pre processor macros that define the maximum
number of tasks that can be created and the size of each task stack respectively.
• The enum state t defines a set of states that a task can be in: running, wait, ready, block, or suspended.
• SysTick Handler – The timer interrupt handler, responsible for updating the delay of waiting tasks
and finding the next ready task with the highestpriority. When a higher-priority task becomes
ready, it sets the next id ready and then triggers the PendSV interrupt with the PendSV call function.
• pendsv Handler – The context switch interrupt handler is responsible for saving the context of
the currently running task (registers R4-R11 and the stack pointer) onto the stack of its TCB,
and then restoring the context of the next task from its TCB. It also updates the state of the
TCBs and the running task ID. Finally, it enables interrupts and returns to the interrupted code with
the special instructions like BX LR instruction. The function does not need to save or restore the
registers R0-R3, PC,XPSR and LR (which are saved automatically by the Cortex-M core), and
that the function has no prologue or epilogue code(which is generated by the compiler by default).
code:
Recent Comments