INTRODUCTION | BLINKY | ASSIGNMENTS |
Task Management
Tasks in FreeRTOS
- With FreeRTOS, an application can be structured as a set of autonomous tasks.
- Each task executes within its own context (e.g., stack) with no coincidental dependency on other tasks.
- The scheduler is responsible for starting, stoping, swapping in, and swapping out tasks.
Tasks Functions
Tasks are implemented as C functions. The prototype of a task function must return void and take a void pointer parameter. A task will typically execute indefinitely in an infinite loop:
- must never terminate by attempting to return to its caller
- If required, a task can delete itself prior to reaching the function end.
A single task function definition can be used to create any number of tasks:
- Each created task is a separate execution instance
- Each created task has its own stack
- Each created task has its own copy of any automatic variables defined within the task itself.
The structure of a typical task function:
void vTaskFunction(void *pvParameters) { int iVariable = 0; /* * Each instance of this task function will have its own copy of the iVariable. * Except that if the variable was declared static – in which case only one copy of the variable * would exist and would be shared by all created instance. */ /* A task will normally be implemented as an infinite loop. */ while(1) { ; /* The code to implement the task functionality will go here. */ } /* * Should the task implementation ever break out of the above loop then the task * must be deleted before reaching the end of this function. The NULL parameter * passed to the vTaskDelete() function indicates that the task to be deleted is the * calling (this) task. */ vTaskDelete( NULL ); }
Task States
An application can consist of many tasks. Only one task of the application can be executed at any given time on the microcontroller (single core). Thus, a task can exist in one of two states:
- Running or
- Not Running.
Only the scheduler can decide which task should enter the Running state. A task is said to have been “switched in” or “swapped in” when transitioned from the Not Running to the Running state, (“switched out” or “swapped out”) when transitioned from the Running state to the Not Running state).
The scheduler is responsible for managing the processor context:
- Registers values
- Stack contents
Task States and Transitions
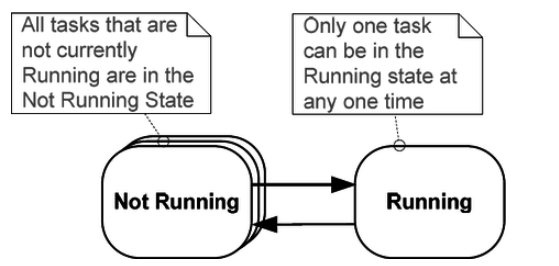
Running state
- The processor is executing its code.
- Not Running state
- The task is dormant, its status having been saved ready for resuming execution the next time
- Scheduler is the only entity that can switch a task in and out a running state.
Creating a Task
A task can be created by calling xTaskCreate() API
portBASE_TYPE xTaskCreate( pdTASK_CODE pvTaskCode, const signed portCHAR * const pcName, unsigned portSHORT usStackDepth, void *pvParameters, unsigned portBASE_TYPE uxPriority, xTaskHandle *pxCreatedTask );
- pvTaskCode
- is a pointer to the function that implement the task
- pcName
- is a descriptive name for the task, not used by FreeRTOS
- configMAX_TASK_NAME_LEN: the application defined constant that defines the maximum length a task name can including the NULL terminator.
- usStackDepth
- specifies the number of words the stack of this task can hold
- E.g., Cortex-M3 stack is 32 bits wide, if usStackDepth is passed in as 100, 400 bytes of stack space will be allocated (100*4 bytes)
- Size of the stack used by the idle task is defined by configMINIMAL_STACK_SIZE.
- pvParameters
- is the parameter that can be passed to the task function (pointer to a complex data structure)
- uxPriority
- is the priority of the task (0 is the lowest priority, configMAX_PRIORITIES-1 is the highest priority)
- Passing a value above (configMAX_PRIOIRTIES -1) will result in the priority being capped the maximum legitimate value.
- pxCreatedTask
- is the handler of the generated task, which can be used to reference the task within API calls
- Returned value
- There are two possible return values:
- pdTRUE
- when the task was created successfully
- errCOULD_NOT_ALLOCATE_REQUIRED_MEMORY
- the task could not be created because there was insufficient heap memory available for FreeRTOS to allocate enough RAM to hold the task data structures and stack.
Expanding the “Not Running” State
- The Blocked state
- A task that is waiting for an event
- Tasks can enter the Blocked state to wait for two different types of events:
- Temporal (time related) events where a delay period expiring or an absolute time being reached
- For example, wait for 10 ms to pass
- Synchronization events where the events originate from another task or interrupt
- For example, wait for data to arrive on a queue
- Temporal (time related) events where a delay period expiring or an absolute time being reached
- It is possible for a task to block on a synchronization event with a timeout
- For example, wait for a maximum of 10 ms for data to arrive on a queue
- The Suspended state
- Tasks in the Suspended state are not available to the scheduler.
- The only way into the Suspended state is through a call to the vTaskSuspend() API function
- The only way out through a call to the vTaskResume() or xTaskResumeFromISR() API functions
- The Ready State
- Tasks that are in the Not Running but are not Blocked or Suspended
- Tasks are able to run, and therefore ready to run, but not currently in the Running state
Task State Transitions
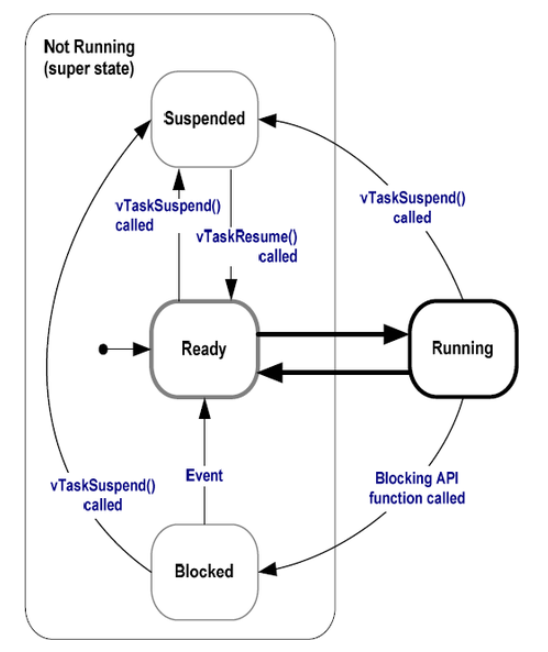
Using the Blocked state to create a delay
- The tasks may generate delay using a null loop – polling a counter until it reaches a fixed value
- Waste of processor cycles
- An efficient method is to create a delay with a call to the vTaskDelay() API function
- vTaskDelay() places the calling task into the Blocked state for a fixed number of tick interrupts
- The task in the Blocked state will not use any processing time at all
- An alternative method is to use the vTaskDelayUntil() API function
vTaskDelay()
void vTaskDelay( portTickType xTicksToDelay );
- xTicksToDelay
- is the number of tick interrupts that the calling task should remain in the Blocked state before being transitioned back into the Ready state.
The constant portTICK_RATE_MS stores the time in milliseconds of a tick, which can be used to convert milliseconds into ticks.
vTaskDelayUntil()
void vTaskDelayUntil(portTickType * pxPreviousWakeTime, portTickType xTicksToDelay );
- pxPreviousWakeTime
- Holds the time at which the task last left the Blocked state (was ‘woken’ up). This time is used as a reference point to calculate the time at which the task should next leave the Blocked state.
- The variable pointed to by pxPreviousWakeTime is updated automatically within the vTaskDelayUntil() function and should be initialized by the application code first.
- xTicksToDelay
- vTaskDelayUntil() is normally being used to implement a task that executes periodically; the frequency being set by this value which is specified in ‘ticks’
Recent Comments