FreeRTOS Exercise Lab21
- Program TIMER0 for 500ms periodic interrupt
- Timer programming How to …
- Create Task 1 as a continuous processing task, and Task 2 (interrupt handler task) as the task to which interrupt processing is deferred.
- In Task 1, display “Task 1 is Running.”
- Display “Timer Interrupt Handler Task is Running” in the Task 2
Note: Task2 should be higher priority than Task1
Code Snippets
/* Task 1 is continuous processing Task */ void vTask1(void *pvParameters) { for(;;) { printf("%s", "Task1 is Running.\n\r"); for(volatile auto uint32_t ul = 0; ul < 0xffff; ul++ ){ ; } } } /* Task 2 as Timer0A Interrupt handler task */ void vTask2(void *pvParameters) { for(;;) { if( xSemaphoreTake(xTimer0ASemaphore, portMAX_DELAY) ) { printf("%s", "Timer Interrupt Handler Task is Running"); } } } /* Timer0A Interrupt Service Routine */ void vTimer0A_ISR(void) { BaseType_t xTaskWoken; TIMER0_ICR_R = 0x01; /* clear Timer0A timeout interrupt */ xSemaphoreGiveFromISR(xTimer0ASemaphore, &xTaskWoken); if(xTaskWoken) { portYIELD_FROM_ISR(xTaskWoken); } }
How it works …
The deferred processing task uses a blocking ‘take’ (xSemaphoreTake(xTimer0ASemaphore, portMAX_DELAY)) call to a semaphore as a means of entering the Blocked state to wait for the event to occur. When the event occurs, the ISR uses a ‘give’ (xSemaphoreGiveFromISR(xTimer0ASemaphore, &xTaskWoken)) operation on the same semaphore to unblock the task so that the required event processing can proceed.
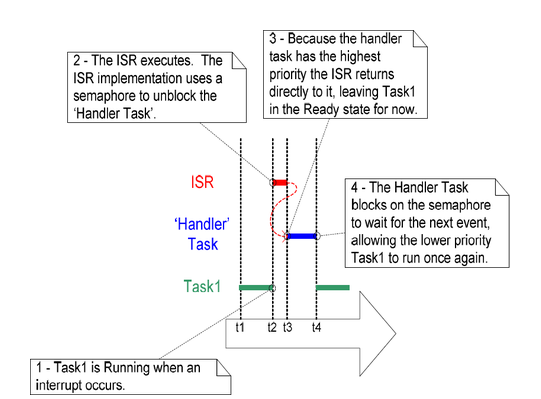
Figure: Using a binary semaphore to implement deferred interrupt processing
Recent Comments