FreeRTOS Exercise Lab20 – Deferred Interrupt Processing
- Create Task 1 as a continuous processing task, and Task 2 (interrupt handler task) as the task to which interrupt processing is deferred.
- In Task 1, display “Task 1 is Running.”
- Install an Interrupt handler for SW1 Button switch (GPIO PortF)
- There are two user push buttons on the EK-TM4c123GXL LaunchPad Board.
- Display “You Pressed SW1 Button switch” in the Task 2 whenever SW1 is pressed
Note: Task2 should be higher priority than Task1
- If the interrupt processing is particularly time critical, then the priority of the deferred processing task can be set to ensure the task always pre-empts the other tasks in the system. The ISR can then be implemented to include a call to portYIELD_FROM_ISR(), ensuring the ISR returns directly to the task to which interrupt processing is being deferred.
Code Snippets
/* Task 1 is continuous processing Task */ void vTask1(void *pvParameters) { for(;;) { printf("%s", "Task1 is Running.\n\r"); for(volatile auto uint32_t ul = 0; ul < 0xffff; ul++ ){ ; } } } /* Deferred interrupt processing Task */ void vTask2(void *pvParameters) { for(;;) { vTaskSuspend(NULL); /* Wait for SW1 interrupt */ printf("%s", "You Pressed SW1 Button switch\n\r"); } } /* PortF Interrupt Service Routine */ void vGPIO_PortF_ISR(void) { BaseType_t xSW1Yield; GPIO_PORTF_ICR_R = 0x10; /* clear PF4 int */ xSW1Yield = xTaskResumeFromISR(hPortF); /* hPortF is Handle of vTask2 */ portYIELD_FROM_ISR(xSW1Yield); }
How It Works …
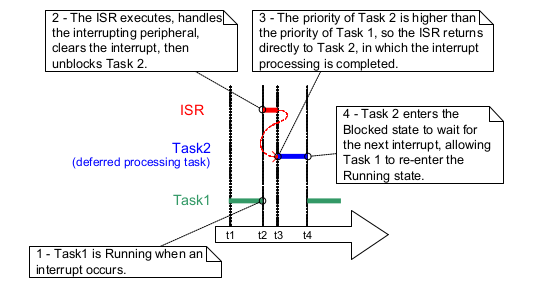
Recent Comments