FreeRTOS Lab 03
- Create a CCS Project using FreeRTOS Project Template
- Add printf-stdarg.c file to project (Project > Add Files …)
- Initialize UART0 for 115,200 baud rate (assuming 16 MHz bus clock), 8 bit word length, no parity bits, one stop bit, FIFOs enabled. (UART0 Echo Source Code is available here)
- Create two tasks at priority 1. These should do nothing other than continuously print out a string.
- These tasks never make any API function calls that could cause them to enter the Blocked state, so are always in either the Ready or the Running state. Tasks of this nature are called ‘continuous processing’ tasks, as they always have work to do.
void vContinuousProcessingTask(void * pvParameters) { char *pcTaskName; pcTaskName = (char *) pvParameters; for (;;) { printf("%s", pcTaskName); vTaskDelay(pdMS_TO_TICKS(100)); } }
- Create a Third task at priority 2.
- Periodically prints out a string by using vTaskDelayUntil() to place itself into the Blocked state between each print iteration.
void vPeriodicTask(void * pvParameters) { portTickType xLastWakeTime; xLastWakeTime = xTaskGetTickCount(); for (;;) { printf("Periodic task is running\n\r”); vTaskDelayUntil(&xLastWakeTime, pdMS_TO_TICKS(20)); } }
- vTaskDelay() does not ensure that the frequency at which they run is fixed, as the time at which the tasks leave the Blocked state is relative to when they call vTaskDelay().
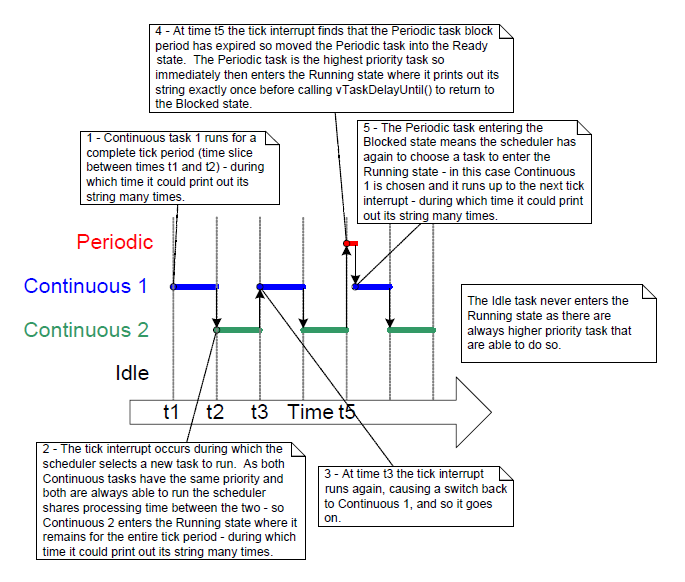
Recent Comments