ABSTRACT
The main aim of this lab experiment is to get familiarized with different way of writing device drivers for the Linux environment. The drivers are supposed to driver LED’s of the traffic light and then also interface Keypad .
I INTRODUCTION
The linux device drivers donot direct interact with the userspace and may of times are part of the kernel module and interacts with user space through API. The interaction is explained in figure 1
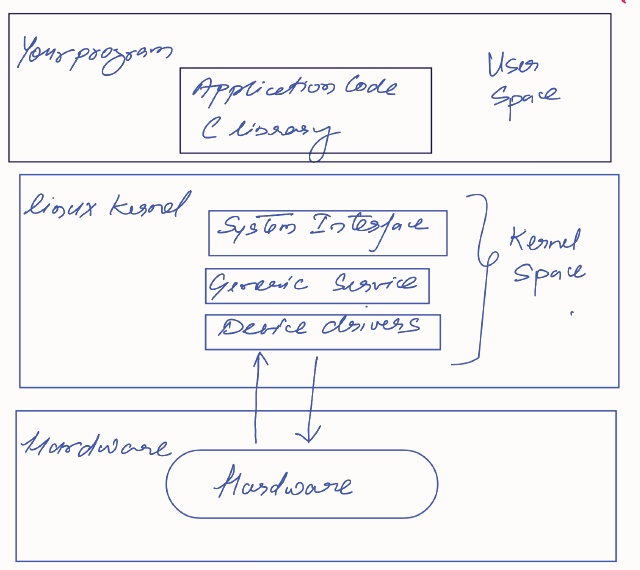
Figure 1: User Space and kernel Space interacting with GPIO hardware
There are two ways of creating driver module:
1. Creating custom code in kernel space and recompiling the kernel
2.Loading the loadable kernel module: this method is more efficient and the module is loaded when its required. Here we are trying the second method and instead of recompiling the whole kernel the developer has to write a LKM( loadable Kernel Module) containg the device driver code.
LKM module can be usefulness in making
• Device Drivers
• File-systems drivers
• System Calls
The linux kernel is written in C and assembly
• C—————> is the main part of the kernel
• Assembly—->Architecture dependent
When working with modules linux links them to kernel by loading them into kernel address space. Care needs to be taken as mistakes can create system level issues. The kernel and its module represent a single program module and use single global namespace.
Character Files: Non buffered files that allow reading and writing data character by character
Block Files: Buffered files that allow to read and write data as whole block.
1.1 File operations: The file operations structure contains pointers to functions defined by the driver. each field of the structure corresponds to the address of a function defined by the driver to handle requested operations.
II IMPLEMENTATION :
Build essentials contain neccessary linux header file and are installed as shown in the figure:
The first method is just controlling each GPIO separately this is done as in the code given below:
#include <linux/module.h>
#include <linux/kernel.h>
#include <linux/gpio.h>
#include <linux/fs.h>
#include <linux/uaccess.h>
#define DEVICE_NAME “gpio_device”
#define GPIO_PIN 17 // Change this to your desired GPIO pin number
static int gpio_open(struct inode *inode, struct file *file) {
// Set GPIO
direction
gpio_request(GPIO_PIN, “GPIO_PIN”);
gpio_direction_output(GPIO_PIN, 0); // Set initial value to low
return 0; }
static ssize_t gpio_write(struct file *file, const char __user *buf, size_t count,
loff_t *offset) {
char kbuf[2];
if (copy_from_user(kbuf, buf, count))
return -EFAULT;
// Set GPIO value based on user input (0 or 1)
gpio_set_value(GPIO_PIN, kbuf
[0] == ’1’ ? 1 : 0);
return count; }
static int gpio_release(struct inode *inode, struct file *file)
{
gpio_free(GPIO_PIN); // Free GPIO pin
return 0; }
static struct file_operations fops = {
.owner = THIS_MODULE,
.open =
gpio_open,
.write = gpio_write,
.release = gpio_release, };
static int __init gpio_driver_init(void) {
register_chrdev(240, DEVICE_NAME, &
fops); // Register device
printk(KERN_INFO “GPIO Driver Loaded\n”);
return 0; }
static void __exit gpio_driver_exit(void) {
unregister_chrdev(240, DEVICE_NAME);
printk(KERN_INFO “GPIO Driver Unloaded\n”); }
module_init(gpio_driver_init); module_exit(gpio_driver_exit); MODULE_LICENSE(“GPL”);
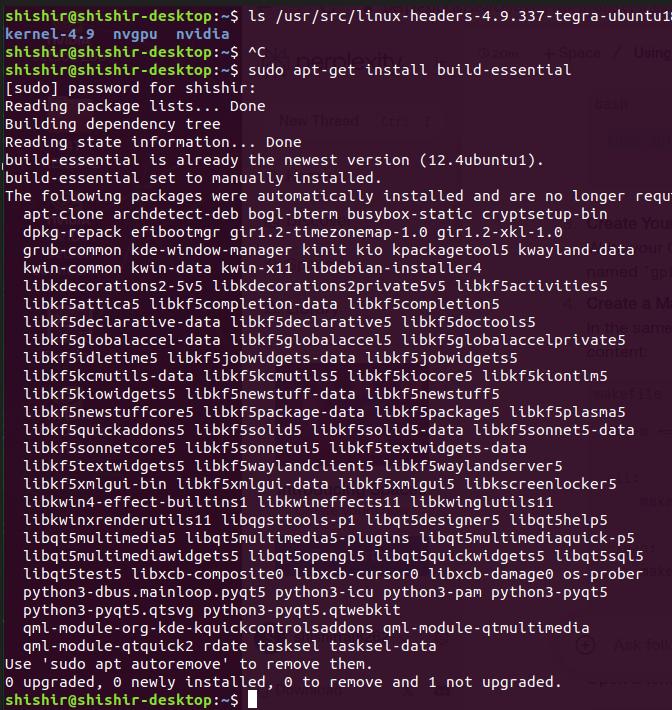
Figure 3: Installation of Device drivers
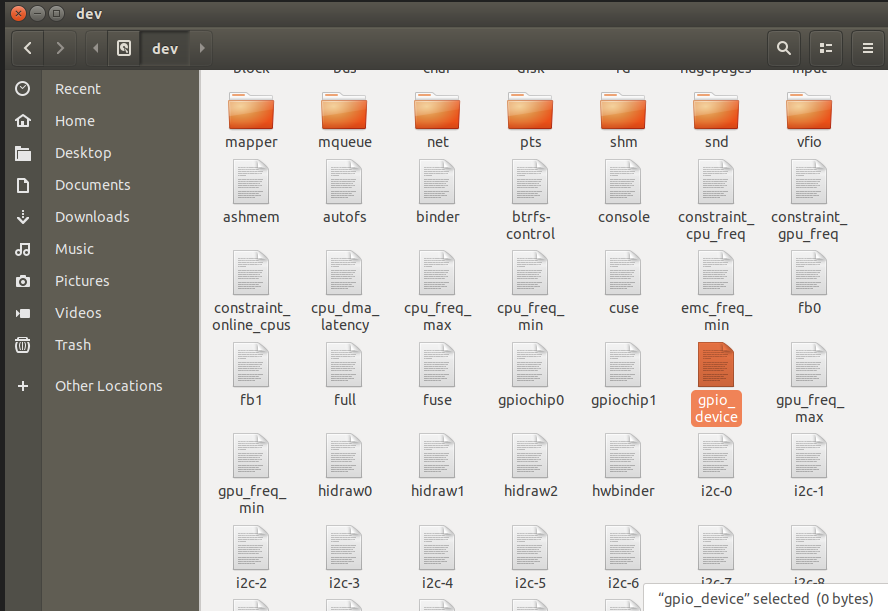
Figure 4: GPIO device created
Using this method the traffic lights can be used using custom kernel module. The proof of the custom GPIO can be seen in the figure …
For creating custom interface for the Keypad it can be done by creating custom gpio class with desired GPIO numbers. This is done inside sys> class. The kernel module has to be loaded as shown in the figure … to unload the module rmmod can be used
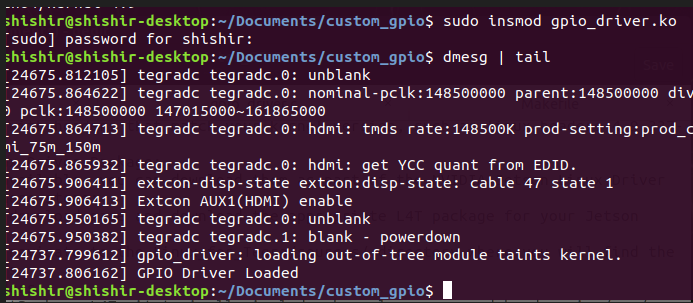
Figure 5: Loading the LKM module
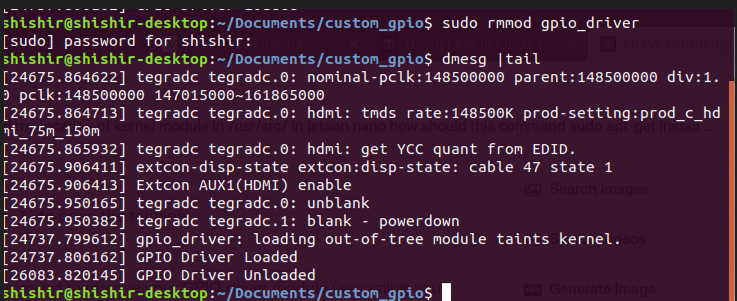
Figure 6: Removing the module
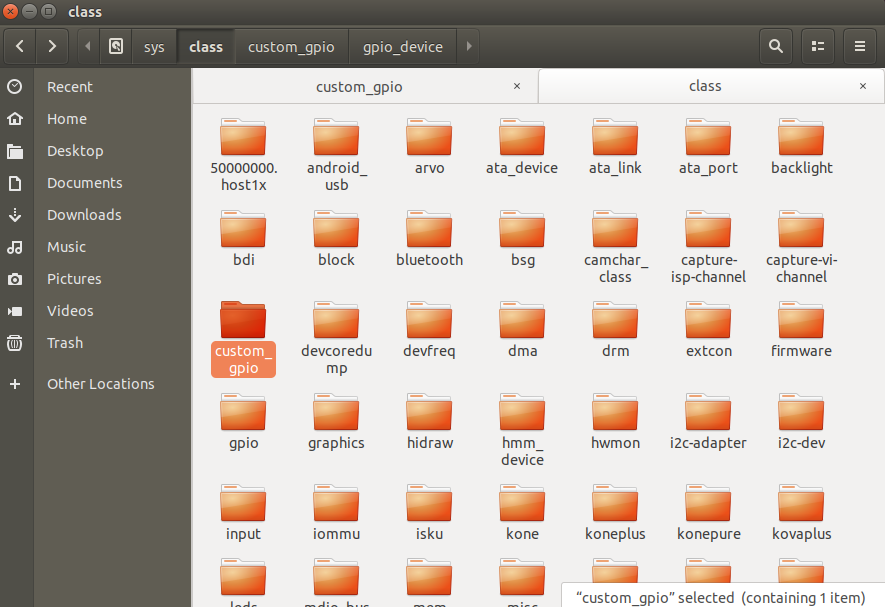
Figure 7:
III OUTPUT AND LESSONS LEARNED :
The custom gpio module was able to act as output but was not functioning as input properly. Also when driving LED the display of Jetson nano was gone. Single LED was getting switched on and off on raspberry pi but keypad could not be interfaced bcm2835 driver was interfering with the function
IV FUTURE SCOPE :
The jetson nano can be interfaced through a buffer to avoid problem of high current sinked/sourced through jetson nano
Recent Comments