Dot LED matrices are a cheap and easy to use hardware. They are commonly used as displaysigns and are often appreciated for their long-range visibility and cost-effective solution for advertising purposes. These Dot LED matrices are configured using MAX 7219 driver. In this project 2 Dot LED matrices are cascaded to display a scrolling text of 4 characters. The user has control over quite a few features of the text which will be discussed in the subsequent sections. The idea is to learn about MAX 7219 driver and use SSI0 hardware built in the processor and the driver to trigger serial communication between the two for data transfers which will be displayed as characters on the Dot LED matrix display.
INITIALISING GPIO PORTF:
GPIO PortF is configured to take inputs from hardware switches that are internally mapped. The SW1 and SW2 switches are connected to PF4 and PF0, respectively. These switches will
be used in the program to trigger interrupts. The GPIO Portf ISR will take of the scroll speed and display intensity. The initialization of Portf for this purpose is mentioned below:
1) Enabling System clock to Portf through SYSCTL_RCGC2_R register.
2) Unlocking Portf through GPIO_PORTF_LOCK_R register.
3) Set the GPIO Commit register to allow changes in Portf through
GPIO_PORTF_CR_R register.
4) Disable the analog mode for Portf in GPIO_PORTF_AMSEL_R register.
5) Set the directions of all the 5 Portf pins using the GPIO_PORTF_DIR_R register, PF4
and PF0 are set as input pins (inputs are set to 0 in the control word).
6) Once the directions are set, pull up resistors are enabled for PF4 and PF0 using
GPIO_PORTF_PUR_R register.
7) Disable the alternate functionality for all pins in Portf using
GPIO_PORTF_AFSEL_R register.
8) Enable digital mode in PF4,PF0 using the GPIO_PORTF_DEN_R register.
9) Portf Interrupt is enabled and configured to sense it at the falling edge.
PORTF INTERRUPT HANDLER:
1) Once SW1 and SW2 are configured to be interrupts, the handler is programmed to detect SW1 and SW2. SW2 controls the intensity of the display. SW1 controls the word
to be displayed.
2) When a particular switch is detected corresponding interrupt flag is cleared and the corresponding check flag is set.
3) The check flag status is checked in the main program in the infinite while loop.
4) When SW1 is pressed, check2 is set and the word being displayed is changed (in other words, global variable index is changed).
5) When SW2 is pressed, check1 is set the Dot matrix is re-initialized with the modified intensity.
INITIALISING UART0:
UART0 is configured to control the Dot Matrix through the UART console. UART0 supports
a set of commands namely,
1) Dim
2) Bright
3) Change
4) Increase/Decrease speed
5) Scroll on/off (only left scroll supported)
The entered key word is matched against the above-mentioned commands and executes the
corresponding task in a function named command_check. UART0 is configured to trigger an
interrupt every time a key is pressed on the keyboard. This is achieved by setting the below
mentioned registers:
1) Enabling clock for UART0 through SYSCTL_RCGCUART_R register.
2) As UART0 Rx and Tx are enabled through Alternate functionality of GPIO Port A,
clock should be enabled for GPIO Port A through SYSCTL_RCGCGPIO_R register.
3) Alternate functionalities of PA0, PA1 are enabled to allow UART0 Rx and Tx, through
GPIO_PORTA_AFSEL_R register.
4) UART0 Tx and Rx are configured to a Baud Rate of 11520, without parity, 1 start bit
and 1 stop bit by setting appropriate registers.
5) UART0 interrupt is enabled in the NVIC and set to a priority of 5.
6) TXIM bit is set in the UART0_IM_R register to detect interrupt on the UART0 Tx
channel.
UART0 INTERRUPT HANDLER:
1) When a key is pressed on the keyboard, an interrupt is triggered.
2) In the interrupt handler, the pressed key is stored in a temporary variable and transmitted back to the console where the character entered is printed.
3) The pressed key is checked if it is an alphabet and stored in a globally defined buffer.
4) When Enter is pressed, check3 flag is set.
5) Inside the while loop of the main program, check3 flag is checked for true condition,when check3 becomes true, the command_check function is called. It checks if the
command (buffer) entered is valid or not and corresponding task is performed.
LED Dot Matrix Display:
In a LED dot matrix display the LEDs are located at the column and row intersections of the matrix. LEDs in the same row are connected together as are LEDs in the same column. Figure
1 shows common cathode configurations for LED matrix display.
MAX7219 Driver
MAX7221 segment drivers are slew-rate limited to reduce electromagnetic interference (EMI),and its serial interface is fully SPI compatible. Initial Power-Up On initial power-up, all control registers are reset, the display is blanked, and the MAX7219 enter shutdown mode.
Program the display driver prior to display use. Otherwise, it willinitially be set to scan one digit, it will not decode data in the data registers, and the intensity
register will be set to its minimum value.

Table 1: Serial-Data Format (16 Bits)
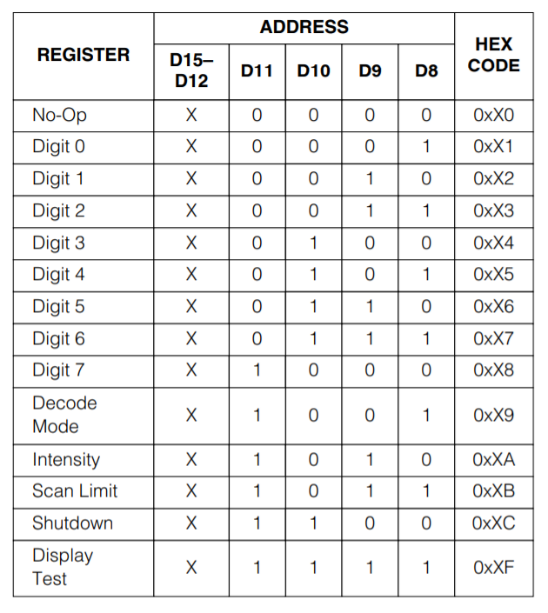
Table 2: Register Address Map
Table 2 lists the 14 addressable digit and control registers. The control registers consist of decode mode, display intensity, scan limit (number of scanned digits), shutdown, and display
test (all LEDs on).
INITIALISING MAX 7219 DRIVER:
STEP 1: Setting up scan limit Register
To select all rows of led dot matrix we set scan register to 0b07.
STEP 2: Setting up Shutdown Register.
Table: Shutdown Register Format (Address (Hex) = 0xXC)
Here MAX7219 is operated in Normal Operation mode.
STEP 3: Setting up Intensity Register
Table: Intensity Register Format (Address (Hex) = 0xXA)
Intensity can be controlled in 16 discrete steps from 0x0 to 0xf.
INITIALISING SSI0:
Step 1: To activate SSI
SYSCTL_RCGCSSI_R |= 0x01;
RCGCSSI: Synchronous Serial Interface Run Mode Clock Gating Control
The RCGCSSI register provides software the capability to enable and disable the SSI modules
in Run mode. When enabled, a module is provided a clock and accesses to module registers
are allowed. When disabled, the clock is disabled to save power and accesses to module
registers generate a bus fault.
Step 2: Activating port A
RCGCGPIO: General-Purpose Input/Output Run Mode Clock Gating Control
The RCGCGPIO register provides software the capability to enable and disable GPIO modules
in Run mode. When enabled, a module is provided a clock and accesses to module registers
are allowed. When disabled, the clock is disabled to save power and accesses to module
registers generate a bus fault.
Step 3: Enabling alternate functionality on PA2,3,5
GPIO_PORTA_AFSEL_R |= 0x2C;
Here PA2 is connected to Clk of MAX7219 Driver and PA3 to CS and PA5 to data in of driver.
Data out of first led matrix is given as data in to next led dot matrix display.
Step 4: Enabling digital Input/Output on PA2,3,5
GPIO_PORTA_DEN_R |= 0x2C;
Step 5: Configure PA2,3,5 as SSI
GPIO_PORTA_PCTL_R = (GPIO_PORTA_PCTL_R & 0xFF0F00FF) + 0x00202200;
GPIO Port Control (GPIOPCTL) register which selects one of several peripheral functions for
each GPIO.
Step 6: Disabling analog functionality on Port A
GPIO_PORTA_AMSEL_R = 0;
Step 7: Disabling SSI
SSI0_CR1_R &= ~SSI_CR1_SSE;
This bit must be cleared before any control registers are reprogrammed.
Step 8: Configuring SSI as Master
SSI0_CR1_R &= ~SSI_CR1_MS;
The SSI is configured as a master.
Step 9: Setting clock polarity and phase to 0
SSI0_CR0_R &= ~(SSI_CR0_SCR_M | SSI_CR0_SPH | SSI_CR0_SPO);
Step 10: Selecting free scale format
SSI0_CR0_R = (SSI0_CR0_R & ~SSI_CR0_FRF_M) + SSI_CR0_FRF_MOTO;
Step 11: Selecting data width
SSI0_CR0_R = (SSI0_CR0_R & ~SSI_CR0_DSS_M) + SSI_CR0_DSS_16;
Step 12: Clock divider for 1.6 MHz SSI Clock (assumes 16 MHz PLL)
SSI0_CPSR_R = (SSI0_CPSR_R & ~SSI_CPSR_CPSDVSR_M) + 10;
Step 13: Enabling SSI
SSI0_CR1_R |= SSI_CR1_SSE;
Programmed SSI0 to Free scale format, 16bit transfer, 0 polarity and 0 phase, clock speed
1.6MHz.
SSI0_WRITE:
Step 1: Disabling Interrupts
Step 2: Choosing SSI0
GPIO_PORTA_DATA_R &= ~0x08;
Step 3: Waiting until FIFO is full and transmitting data through SSI
while ((SSI0_SR_R & 2) == 0); /* wait until FIFO not full */
SSI0_DR_R = data; /* transmit high byte */
Step 4: Waiting until transmission is done by monitoring status register
while (SSI0_SR_R & 0x10); /* wait until transmit complete */
GPIO_PORTA_DATA_R |= 0x08; /* keep SS idle high */
Step 5: Enabling Interrupts
SOFTWARE SETUP
GPIO PortF, UART0, SSI0 and the Dot matrix drivers are initialized and the characters to be
displayed are globally defined. Once the strings to be displayed are defined, the characters are identified and the related data are stored in separate buffers. All of this is done in create_encoded_data function. These buffers contain the row data of all characters. In the main program, scrollstring function is called in a while loop. Inside the scrollstring function, scroll flag is checked for true condition. If scroll flag is true, the row data is shifted and stored in globally defined encoded_data matrix. The encoded_data is sent through SSI0_write function. To control the speed of scroll, a global variable is defined and the same data is printed in a for loop controlled by this variable. If the scrolling speed must be increased, the speed variable is reduced so that same data is printed lesser number of times.
While the above mentioned loop goes on, UART0 and PortF interrupts are set up to give user more control over scroll speed, brightness etc. When SW1 or SW2 is pressed, Portf interrupt
handler is called. SW1 increases the brightness. When the brightness reaches maximum level, it is reset to minimum level in this handler. Similarly when SW2 is pressed, the index variable
is changed and the characters being displayed are changed. UART0 interrupt handler is set up to give greater control. From UART, user can give 7 commands which controls various features of display. If user enters the command “dim” intensity is reduced by 5 whereas if “bright” is entered, intensity is increased by 5. If “change” is entered, the characters being displayed will change. If “scroll on” is entered, the characters will scroll left infinite number of times whereas “scroll off” will stop scrolling altogether. “Increase speed” will decrease the value of speed variable, increasing the scrolling speed
whereas “decrease speed” will increase the value of speed variable, decreasing the scrolling speed. If any other command is entered, the programs prints “Invalid command” and prints the
list of commands supported in the program.
RESULTS:
console commands:
FUTURE SCOPE
1) Program can be modified to enable scrolling option as well, like “scroll left” and “scrollright”
2) The use of DMA in this program to copy the data to SSI data register periodically is an avenue to be explored. Using DMA will make the execution much faster.
3) The program is restricted to print only 4 characters on the 2 Dot displays we have. The program can be extended to displaying more than 4 characters.
REFERENCES
[1] MAX7219 datasheet
[2] TIVA C SERIES datasheet
*Datasheets are attached in project folder
Demo video
Recent Comments